To set up an API endpoint using PHP and mySQL to receive iOT data, follow these steps:
Suggested Read – What are APIs and how do APIs work?
Set up your web server
Create mySQL database
Create a PHP script to handle incoming data
Create a PHP script that will handle the incoming iOT data and store it in the mySQL database. Here’s an example script that accepts POST requests and inserts the data into a mySQL database:
<?php$servername = “localhost”;
$username = “yourusername”;
$password = “yourpassword”;
$dbname = “yourdatabase”;// Create connection
$conn = new mysqli($servername, $username, $password, $dbname);// Check connection
if ($conn->connect_error) {
die(“Connection failed: ” . $conn->connect_error);
}// Get the POST data
$data = file_get_contents(‘php://input’);
$data = json_decode($data, true);// Insert data into mySQL database
$sql = “INSERT INTO iot_data (timestamp, temperature, humidity)
VALUES (’{$data[‘timestamp’]}‘, ’{$data[‘temperature’]}‘, ’{$data[‘humidity’]}’)“;if ($conn->query($sql) === TRUE) {
echo “Data inserted successfully”;
} else {
echo “Error: ” . $sql . “<br>” . $conn->error;
}$conn->close();
?>
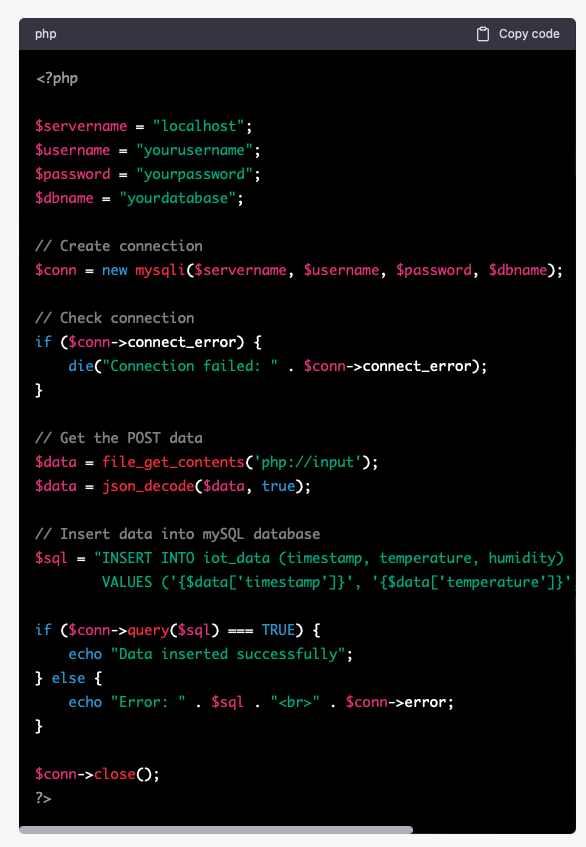
iot_data
table in the mySQL database.
Schedule a free consultation today
Want to speak to a human about how integrating system softwares via an API? Fill out the form below for a free consultation.
Set up the API endpoint
To set up the API endpoint, save the PHP script from step 3 on your web server and define a route to access it. For example, if you save the script as iot.php
, you can define a route like this:
<?phpif ($_SERVER[‘REQUEST_METHOD’] == ‘POST’) {
include(‘iot.php’);
} else {
// Handle other HTTP methods here
}?>
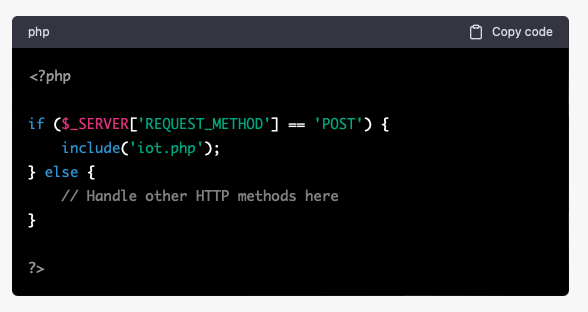
This code checks if the request method is POST and includes the iot.php
script if it is.
Send data to the API endpoint
Here’s an example cURL command:
curl –location –request POST ‘https://yourdomain.com/api/iot’ \
–header ‘Content-Type: application/json’ \
–data-raw ‘{
“timestamp”: “2022-03-30 13:00:00″,
“temperature”: 25,
“humidity”: 60
}’
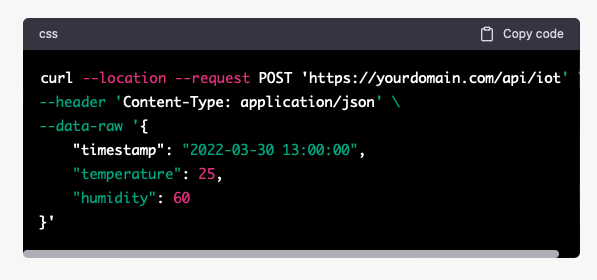
This command sends a POST request to the https://yourdomain.com/api/iot
endpoint with JSON data containing a timestamp, temperature, and humidity.
In conclusion…
In summary, to set up an API endpoint using PHP and mySQL to receive iOT data, you need to create a mySQL database, create a PHP script to handle incoming data, define a route to access the script, and send data to the API endpoint using a tool such as Postman or cURL. By following these steps, you can create an API endpoint that receives iOT data and stores it in a mySQL database.
Need more information on setting up an API endpoint using PHP and mySQL? Give us a call on 303.473.4000 or click here to get in touch.
Stay tuned for more…
Jeff
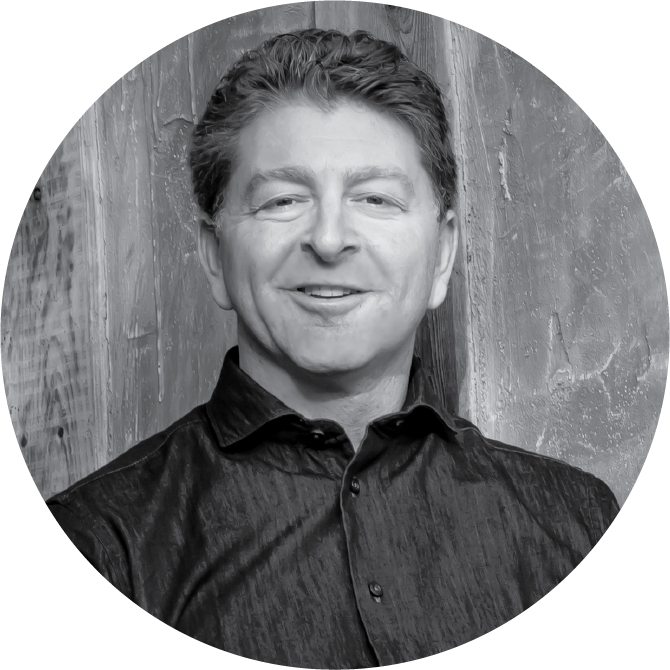
Busy running your business? We can help..
Our team of programmers are here to help you with all of your API system integration needs. Click here to start your free consultation today.
READY TO START GROWING YOUR BUSINESS?
Schedule a free, No Obligation Consultation about our Digital Marketing Services
let’s start marketing
Say Hello!
We would love to discuss your project with you. Get in touch by filling out the form below and we’ll contact you asap. Want to speak to a human now? Text or call 303.473.4400